We are thrilled to announce an exciting new blog series dedicated to exploring and understanding the fascinating world of algorithms. Whether you're a budding programmer, a seasoned developer, or someone simply curious about the intricacies of coding, this series is designed to cater to all levels of expertise.
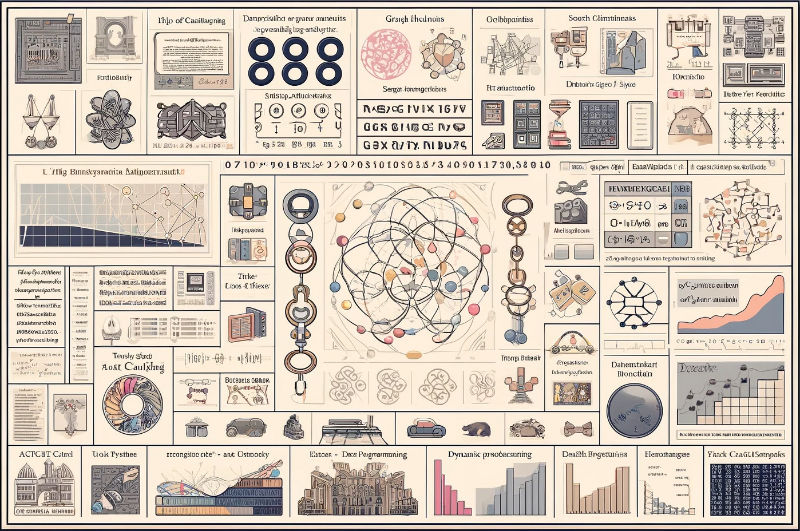
What to Expect from the Series
Each blog post in this series will introduce a specific algorithm, delving deep into its core concepts, applications, and real-world significance. We will provide a detailed description of how each algorithm works, followed by a step-by-step guide to implementing it in Python. Here's a sneak peek of what you can look forward to:
1. Introduction to Algorithms:
Understanding what algorithms are and why they are important.
The role of algorithms in computer science and everyday applications.
2. Sorting Algorithms:
Bubble Sort, Merge Sort, Quick Sort, Insertion Sort, Selection Sort, and Heap Sort.
Detailed explanations and Python code implementations.
3. Search Algorithms:
Linear Search, Binary Search, Interpolation Search, and Exponential Search.
Use cases and Python code examples.
4. Graph Algorithms:
Depth-First Search (DFS), Breadth-First Search (BFS), Dijkstra's Algorithm, A* Search Algorithm, Bellman-Ford Algorithm, Floyd-Warshall Algorithm, and Kruskal's and Prim's Algorithms for Minimum Spanning Trees.'
Graph Traversal and Shortest Path Algorithms: Algorithms for finding the shortest path in graphs, including Dijkstra's, Bellman-Ford, and Floyd-Warshall.
Practical applications and Python coding tutorials.
5. Dynamic Programming:
Understanding the concept of dynamic programming.
Solving problems like the Knapsack Problem, Longest Common Subsequence, Fibonacci Sequence, and Matrix Chain Multiplication with Python.
6. Greedy Algorithms:
Introduction to greedy techniques.
Examples and Python code for problems like the Huffman Coding, Activity Selection, and the Coin Change Problem.
7. Backtracking Algorithms:
Solving puzzles and problems using backtracking.
Coding examples like the N-Queens problem, Sudoku Solver, and Hamiltonian Path in Python.
8. Divide and Conquer Algorithms:
Understanding the divide and conquer approach.
Examples and Python code for algorithms like Merge Sort, Quick Sort, and the Fast Fourier Transform (FFT).
9. String Algorithms:
String matching algorithms like Knuth-Morris-Pratt (KMP), Rabin-Karp, and Boyer-Moore.
Examples and Python implementations for problems like Longest Common Substring and String Search.
10. Tree Algorithms:
Traversal algorithms like Inorder, Preorder, Postorder, and Level Order.
Binary Search Trees, AVL Trees, and Segment Trees.
Practical applications and Python coding tutorials.
11. Hashing Algorithms:
Understanding hash functions and hash tables.
Examples and Python code for implementing a hash table and solving hash-based problems.
12. Mathematical Algorithms:
Algorithms for GCD, LCM, Prime Factorization, Sieve of Eratosthenes, and Fast Exponentiation.
Python code implementations and applications.
Why You Should Follow This Series
Comprehensive Learning: Each blog will not only explain the algorithm but also provide practical coding examples, helping you to understand and implement the concepts effectively.
Hands-On Experience: By following along with the Python implementations, you'll gain valuable hands-on experience that will enhance your coding skills.
Real-World Applications: Learn how these algorithms are applied in various domains, from web development to artificial intelligence.
Community and Support: Join a community of learners, ask questions, share your progress, and get support from peers and experts.
Subscribe and Stay Updated
We invite you to subscribe to our blog and be a part of this educational journey. By subscribing, you'll receive notifications for each new post in the series, ensuring you never miss out on any valuable content.
To subscribe, simply enter your email address in the subscription box below or follow us on our social media channels for updates.
Let's embark on this algorithmic adventure together and unlock the full potential of your coding capabilities!
Happy Coding!
Comments