Creating a game in Pygame that involves simulating the trajectory of a ball based on different gravitational forces on different celestial bodies is quite complex. It requires using calculus to calculate the path of the ball as it moves through space, taking into account the varying gravitational pull of each celestial body.
Imagine trying to throw a ball on Earth, where gravity pulls it down in a predictable arc. Now, imagine trying to throw that same ball on the Moon or Mars, where the gravity is weaker or stronger than on Earth.
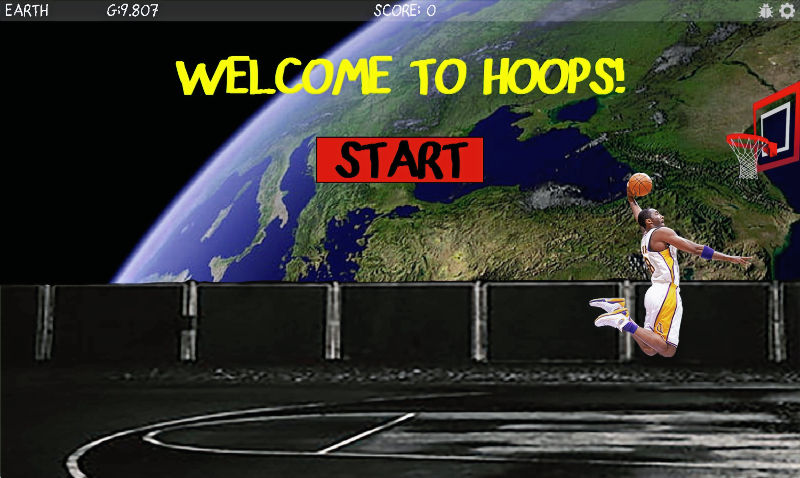
To simulate this in Pygame, we need to calculate the trajectory of the ball using formulas that take into account the gravitational force of each celestial body. This involves complex mathematical calculations that determine how the ball moves and interacts with the environment in the game.
Different celestial bodies
Calculating ball trajectory using calculus
The calc_trajectory method calculates the trajectory of a ball based on the initial click position (starting_ball_pos) and the current mouse position (pos). First, it determines the slope of the line between the two points, accounting for vertical lines. Then it calculates the speed of the ball as the Euclidean distance between the points. Using the slope, it calculates the angle of the trajectory.
The method adjusts the angle based on the quadrant in which pos lies relative to starting_ball_pos. It returns the trajectory path using the calculated speed and angle.
def calc_trajectory(self, pos):
# calculate slope
if (self.starting_ball_pos[0]-pos[0]) != 0:
slope = (self.starting_ball_pos[1]-pos[1]) / \
(self.starting_ball_pos[0]-pos[0])
else:
slope = math.inf
# Using distance as a proxy for speed
speed = math.sqrt((self.starting_ball_pos[1]-pos[1])*(self.starting_ball_pos[1]-pos[1]) + (
self.starting_ball_pos[0]-pos[0])*(self.starting_ball_pos[0]-pos[0]))
# tan(θ) = slope, atan returns arc tangent of slope as a numeric value between -PI/2 and PI/2 radians (i.e. ±1.57)
angle = math.atan(slope)
# adjust angle for right two quadrants
if pos[0] > self.starting_ball_pos[0] and pos[1] >= self.starting_ball_pos[1]:
# clicking in bottom right quadrant
angle = angle + (math.pi)
elif pos[0] > self.starting_ball_pos[0] and pos[1] < self.starting_ball_pos[1]:
# clicking in top right quadrant
angle = angle - (math.pi)
return self.get_path(self.starting_ball_pos, speed * math.cos(angle), speed * math.sin(angle))
I loved this game challenge as it required deep understanding of physics, mathematics, and programming to accurately simulate the behavior of the ball in different gravitational conditions.
Here is the sneak peek of the final game:
Kommentarer