You are just learning your first commands of python. The print() command. The input() command. Creating your own variables. Eventually adding some logic here and there. But you are just not understanding, what's the point of all of this? What can I actually do with python? Today, we will be diving into some ultra simple and fun python projects, to help you answer these questions!
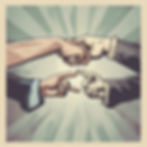
Project 1. Rock Paper Scissors Game (9 lines of code!)
The traditional Rock Paper Scissors Game that we all enjoy playing for fun and having it be a random decision maker for us! Did you know you can play rock paper scissors on the computer?
Start of by typing. - The blue text will represent code
import random
Random is a python library used to simply make random choices!
Next we need an input from the player and have the input be stored in a variable to be used for logic later.
player = input("What is your choice - Rock, Paper, or Scissors?")
The player has made their decision, what about the computer? We can execute a command using the random library to choose a random value from a list. In this list we will put the string values "rock" "paper" "scissors" The random choice will be stored in the variable as the computer's choice for logic later. Then using the format command (used to print variables), we can print the computers choice.
computer = random.choice(["rock","paper","scissors"])
print(f"The computer's choice is {computer}")
Just some logic comparing the variables and then we are done, this part is very intuitive, just be careful to have the correct syntax!
if player == computer: print("It is a tie") Many people like to put the print() under the if statement but it doesn't matter! You can type the print() after the if statement
Similarly . . .
elif player == "rock" and computer == "scissors": print("You Win!")
elif player == "scissors" and computer == "paper": print("You Win!")
elif player == "paper" and computer == "rock": print("You Win!")
else: print("You lost . . . Please, play again!")
Project 2. Mad Libs (5+ lines of code!)
The all classic mad libs game that makes everyone laugh, it's surprisingly really easy to make using python!
Start of by defining as many variables as you want that are inputs from the user!
adjective = input("Enter an adjective: ")
verb = input("Enter a verb:")
job = input("Enter a job: ")
favorite_class = input("Enter your favorite class: ")
Mash it all together into your story! Remember we need to use the format command so we can print the variables.
print(f"Coding is so {adjective}! It makes me want to {verb}. One day I want to be a {job} and my favorite class at school is {favorite_class}.")
Feel free to customize this as much as you want!

Project 3. Grade Calculator (9 lines of code!)
You get your math test's score back from school, but you've just had a tiring day and just aren't able to mentally calculate your percentage and grade. Why not create a grade calculator using python?
Let's start off by declaring the score variable and having a it store a value of the user's test score. When a computer receives an input from the user, it always comes as a string data type. Even if it's a number. Since we need to make some calculations and do some logic with numbers, we need to the input convert to a float data type by using float() command. Simply said - it's converting the input to a decimal.
grade1 = float(input("Your score:"))
Next we need to see how many points the test was out of:
grade2 = float(input("Out of how many points?"))
Time to find the user's percent on their test! We can simply write an expression like we do in math and the computer usually understands it, as long as the variables in the expression hold number data types like floats and integers. After that we will print it out again - using format and print().
grade = (grade1/grade2)*100
print(f"{grade}%")
Just add this intuitive logic and we are done! You may adjust the intervals for the grade to be categorized as an A or B etc.
if grade >= 90: print("You received an A!")
elif grade >= 80: print("You received a B")
elif grade >= 70: print("You received a B")
elif grade>=60: print("You received a D")
elif grade<60: print("You received an F")
If the % was 95 you might think the computer should print all 5 commands. But since logic is read from top-to-bottom, when it reaches the first true statement, it the computer stops reading the rest. That's why only You received an A!" should be printed!
That's it for now! Keep an eye out - I will write many more awesome computer-programming projects you can do, not just for beginners, but for advanced programmers too!