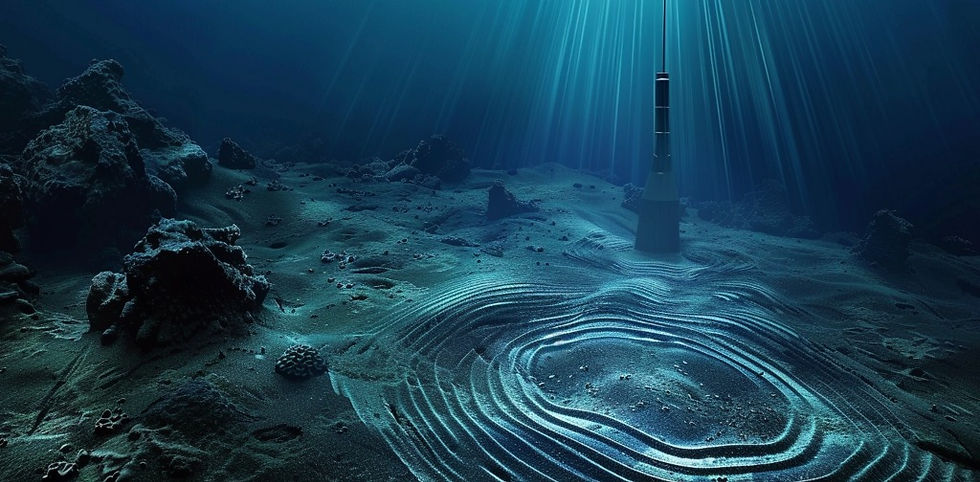
SONAR is Sound Navigation and Ranging. It’s a technique that uses sound propagation to navigate, communicate with or detect objects on (or under) the water surface, such as other vessels.
In this blog, we will go through the steps of how to build a Sonar system using an Ultrasonic Sensor and Arduino.
Hardware
Name | Quantity | Component |
D1 | 1 | Red LED |
D2 | 1 | Green LED |
U1 | 1 | Arduino Uno R3 or Arduino MEGA |
PING1 | 1 | Ultrasonic Distance Sensor |
PIEZO1 | 1 | Piezo / Buzzer |
D3, D4 | 2 | Diode |
R2 | 1 | 100 ohm Resistor |
Connections: Setup the Ultrasonic Sensor
Connect the VCC pin of the Ultrasonic Sensor to the 5V pin on the Arduino. The circuit below shows how it uses a breadboard to streamline 5V and ground connections
Connect the GND pin of the Ultrasonic Sensor to any GND pin on the Arduino.
Connect the SIG pin of the Ultrasonic Sensor to digital pin A1 on the Arduino.
Connect Piezo / Buzzer via register to GND
Connect Piezo / Buzzer via a diode to green LED and also to red LED
Connect LEDs ground to GND
Connect green LED +ve to pin 13
Connect red LED +ve to pin 12
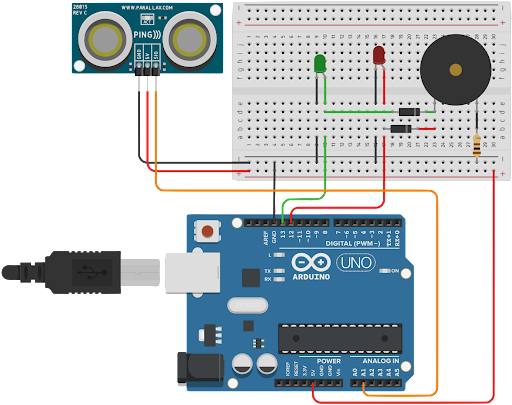
Coding the Arduino: Sketch
Defines two notes, NOTE_C4 and NOTE_A3, with their corresponding frequencies
Define a function readUltrasonicDistance to measure the distance using an ultrasonic sensor connected to a specified pin.
long readUltrasonicDistance(int pin) {
// Clear the trigger
pinMode(pin, OUTPUT);
digitalWrite(pin, LOW);
delayMicroseconds(2);
// Sets the pin on HIGH state for 10 microseconds
digitalWrite(pin, HIGH);
delayMicroseconds(10);
digitalWrite(pin, LOW);
pinMode(pin, INPUT);
// Reads the pin, and return the sound wave travel time in ms
return pulseIn(pin, HIGH);
}
In the setup function, initialize the serial communication and sets two pins (12 and 13) as outputs.
void setup() {
pinMode(A1, INPUT);
Serial.begin(9600);
pinMode(12, OUTPUT);
pinMode(13, OUTPUT);
}
In loop() function, continuously read the distance from the ultrasonic sensor using our readUltrasonicDistance function, convert it to centimeters, and print it to the serial bus. If the distance is less than 40 cm, light up one LED (pin 12), turn off the other LED (pin 13), and play a tone corresponding to the NOTE_A3 frequency.
If the distance is greater than 40 cm, light up the other LED (pin 13), turn off the first LED (pin 12), and play a tone corresponding to the NOTE_C4 frequency.
void loop() {
Serial.println(0.006783 * readUltrasonicDistance(A1));
if (0.006783 * readUltrasonicDistance(A1) < 40) {
digitalWrite(12, HIGH);
digitalWrite(13, LOW);
beep(12, NOTE_A3);
} else {
digitalWrite(13, HIGH);
digitalWrite(12, LOW);
beep(13, NOTE_C4);
}
// Delay a little bit to improve simulation performance
delay(10);
}
Beep function based on the pin and note, used in the loop() above
void beep(int pin, int note) {
// calculate note duration, take 1s divided by the note type.
// e.g. quarter note = 1000 / 4, eighth note = 1000/8, etc.
int noteDuration = 1000 / 2;
tone(pin, note, noteDuration);
// to distinguish the notes, set a minimum time between them.
// the note's duration + 30% seems to work well:
int pauseBetweenNotes = noteDuration * 1.30;
delay(pauseBetweenNotes);
// stop the tone playing:
noTone(pin);
}
Building a Sonar system using an Ultrasonic Sensor is a fun and educational and allows us to understand the principles of sonar.
Comments